게임 개발을 공부하면 오브젝트 풀에 대해 배우면서
오브젝트를 미리 생성하고 필요할때 꺼내서 사용하고 사용이 다되면 풀에 반납하여 비활성화 시켜주는 것을
오브젝트 풀링이라고 배워왔다.
하지만 유니티에서는 이 오브젝트 풀을 사용 할수 있게 패키지로 제공하고 있다.
using UnityEngine.Pool;
해당 패키지를 사용하기 위해서는 해당 코드를 작성하고 사용해야된다.
해당 Pool를 사용하려면 어떡해 해야되는가?
public IObjectPool<GameObject> objectPool;
public ObjectPool<GameObject> pool;
변수로 선언할때는 이 두방식을 이용하여 변수를 초기화 하여 사용하면된다.
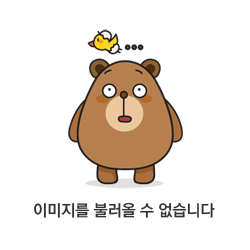
pool = new ObjectPool<GameObject>(CreateObject, GetObject, ReleaseObject, DestroyObject, collectionChecks, minSize, maxSize);
objectPool = new ObjectPool<GameObject>(CreateObject, GetObject, ReleaseObject, DestroyObject, collectionChecks, minSize, maxSize);
이런식으로 선언을 하면된다.
namespace UnityEngine.Pool
{
public interface IObjectPool<T> where T : class
{
int CountInactive { get; }
T Get();
PooledObject<T> Get(out T v);
void Release(T element);
void Clear();
}
}
해당 코드가 IObjectPool의 내부 구조이다.
using System.Collections.Generic;
using System.ComponentModel;
using UnityEditor.Presets;
using UnityEngine;
using UnityEngine.Pool;
//최소 50개의 오브젝트 수 보장, 부족할 경우 누적 300개까지 추가 생성, 300개가 넘어갈 경우 가장 오래전에 생성된 오브젝트를 반환 후 재사용
public class Week2_OjbectPool_Q4 : Singleton<Week2_OjbectPool_Q4>
{
[SerializeField] private GameObject prefab;
public ObjectPool<GameObject> pool;
public bool collectionChecks = true;
private const int minSize = 50;
private const int maxSize = 300;
private GameObject container;
void Awake()
{
container = new GameObject(prefab.name + "_Container");
/*
createFunc: 오브젝트 생성 함수 (Func)
actionOnGet: 풀에서 오브젝트를 가져오는 함수 (Action)
actionOnRelease: 오브젝트를 비활성화할 때 호출하는 함수 (Action)
actionOnDestroy: 오브젝트 파괴 함수 (Action)
collectionCheck: 중복 반환 체크 (bool)
defaultCapacity: ObjectPool의 List<T>에 미리 자리 만드는것(오브젝트 생성x)(int)
maxSize: 저장할 오브젝트의 최대 갯수 (int)
*/
pool = new ObjectPool<GameObject>(CreateObject, GetObject, ReleaseObject, DestroyObject, collectionChecks, minSize, maxSize);
}
private GameObject CreateObject()
{
// [요구스펙 1] Create Object
//Instantiate();
GameObject obj = Instantiate(prefab, container.transform);
return obj;
}
public void GetObject(GameObject obj)
{
// [요구스펙 2] Get Object
obj.gameObject.SetActive(true);
}
public void ReleaseObject(GameObject obj)
{
// [요구스펙 3] Release Object
obj.gameObject.SetActive(false);
}
public void DestroyObject(GameObject obj)
{
//오브젝트 파괴 함수
Destroy(obj.gameObject);
}
private void Update()
{
if (Input.GetKeyDown(KeyCode.Space))
{
for (int i = 0; i < 10; i++)
{
pool.Get();
}
}
}
}
해당 코드는 UnityEngine.Pool을 이용하여 오브젝트 풀을 구성한 예제 코드이다.
사용시에는 따로 풀링할 오브젝트를 넣어 등록해서 사용해야된다.
해당 Docs를 참고하여 글을 작성하였습니다.
https://docs.unity3d.com/ScriptReference/Pool.ObjectPool_1.html
Unity - Scripting API: ObjectPool<T0>
Object Pooling is a way to optimize your projects and lower the burden that is placed on the CPU when having to rapidly create and destroy new objects. It is a good practice and design pattern to keep in mind to help relieve the processing power of the CPU
docs.unity3d.com
'Unity' 카테고리의 다른 글
Unity :: 셰이더 그래프 (0) | 2025.03.11 |
---|---|
Unity :: Rigidbody컴포넌트의 Rigidbody.AddForce() , ForceMode (0) | 2024.10.24 |
Unity :: (2024.10.21수정)제네릭 클래스를 상속받은 Class는 오브젝트 생성 및 컴포넌트로 사용 할수 잇지만 제네릭 클래스 그 자체는 사용 할 수 없다. (0) | 2024.10.21 |
Unity :: TMP(Text Mesh Pro) 한글 폰트로 쓰기 , 스크립트에서 사용하기 (0) | 2024.10.15 |
Unity :: 비트연산자와 레이어마스크 (0) | 2024.10.14 |