https://leetcode.com/problems/flood-fill/description/
문제 해결 일자 : 2024.09.24
난이도 : easy
문제 풀이 / 코드 구현 : 직접 구현 / 직접 구현
더보기
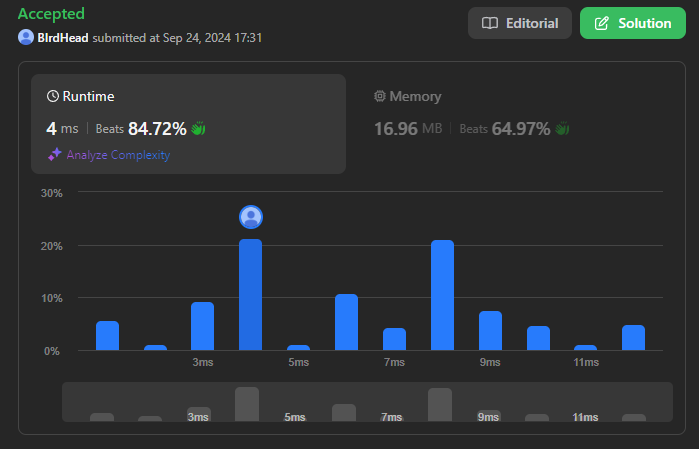
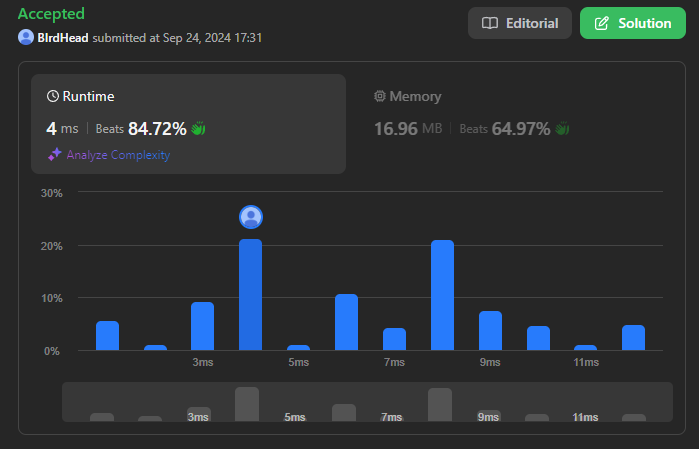
카테고리 : 배열, DFS , BFS , Matrix
문제 내용 :
좌표(sr,sc)한곳을 시작하여 인접하고 있는 좌표들의 value값을 바꾸는 문제이다.
이때 인접은 상,하,좌,우만 해당된다.
문제 풀이 :
시작 좌표가 주어지고 자기 자신과 다른 value값을 가졌으면 이동하지 않으면 되고 방향(상,하,좌,우)가 정해졌기에 재귀함수로 접근하여 문제를 풀었다.
접근 순서:
1.시작 좌표의 value값을 변경(1 -> 2)한다.
2.방향 순서로 한칸 이동한다.
3.범위를 벗어났거나 처음Value값(1)이 다르면 돌아온다 같은경우 해당 방향으로 한칸 더 이동한다.
4. 2 - 3을 방향값을 변경하며 다 변경 될때까지 반복한다.
구현코드
더보기
#include <iostream>
#include <vector>
using namespace std;
class Solution
{
public:
enum dir { UP = 1, DOWN = -1, LEFT = -1, RIGHT = 1 };
void FindPixel(vector<vector<int>>& image, int sr, int sc, int pos,int color)
{
if((sr < 0 || sr >= image.size()) || (sc < 0 || sc >= image[0].size()))
{
return;
}
if (image[sr][sc] != pos)
{
return;
}
image[sr][sc] = color;
FindPixel(image, sr + UP, sc, pos, color);
FindPixel(image, sr + DOWN, sc, pos, color);
FindPixel(image, sr, sc + LEFT, pos, color);
FindPixel(image, sr, sc + RIGHT, pos, color);
}
vector<vector<int>> floodFill(vector<vector<int>>& image, int sr, int sc,int color)
{
if (image[sr][sc] == color)
{
return image;
}
FindPixel(image, sr, sc, image[sr][sc], color);
return image;
}
};
'코딩테스트 > LeetCode' 카테고리의 다른 글
LeetCode :: 300. Longest Increasing Subsequence (C++) (0) | 2024.09.25 |
---|